IDNZ AI Assistant
Developed a custom AI assistant using an OpenAI framework to take course inquiries and direct potential clients to consultation services for the Institute of Digital Marketing New Zealand with a React interface, Node.js middleware, and database integration. Implemented bidirectional iframe communication for Squarespace embedding, two-step API architecture for course information retrieval, and HubSpot REST API integration for lead capture functionality.
Main Takeaways
- Implemented function calling with OpenAI API to create context-aware conversations that dynamically load relevant data
- Engineered a cross-domain communication system between iframes and parent windows using postMessage API and event listeners
- Designed an architecture that reduced API token usage by 73% through strategic prompt engineering and caching mechanisms
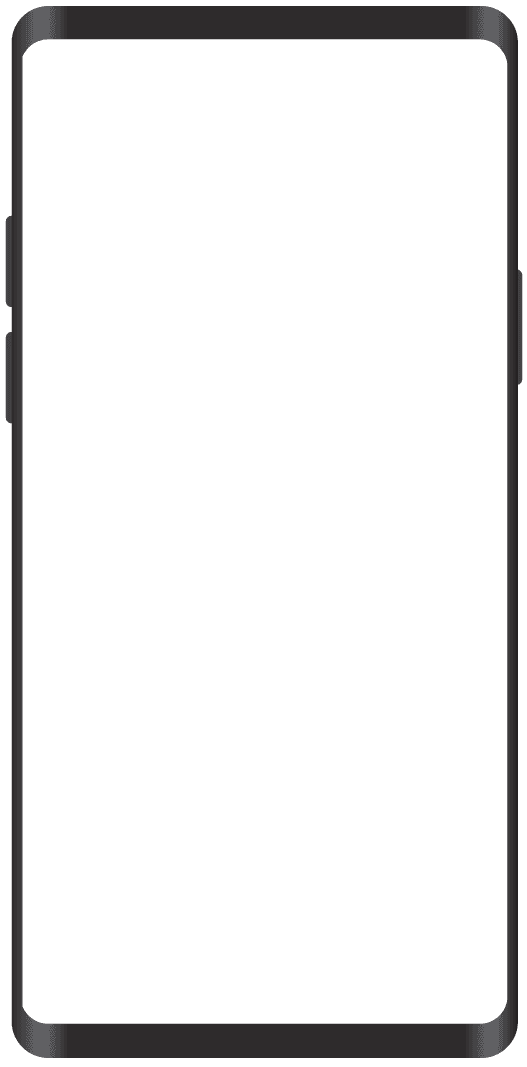
Try it out here
Design and Discussions
The first step was designing the chatbot interface using React components with custom hooks for state management. A key element was enabling the bot to toggle from a large size to a minimized size, which was challenging since it was injected through an iframe in Squarespace. To solve this, I implemented a bidirectional communication protocol using the postMessage API and added event listeners to the embedded code so it could expand or contract the chatbot on the main page. The team at IDNZ had initially asked if we could integrate it with ManyChat, but I explained the limitations of prebuilt services, particularly regarding API rate limiting and customization constraints. We ultimately decided that I would write our own application using TypeScript and Next.js, which would give us better control over the implementation, and allow for a more responsive chatbot at a lower cost.
OpenAI API Infrastructure
I built a Next.js API route utilizing OpenAI's function calling for efficient course information retrieval. My implementation used a two-step approach where the first API call evaluates user queries, and if course details are needed, triggers a second call that fetches only the relevant information. I implemented course-specific prompts, maintained conversation context between calls, and added comprehensive logging for debugging. Using GPT-4o-mini with carefully tuned temperature settings gave us the right balance of creativity and accuracy while keeping costs manageable for the client.
async function getCourseDetails(courseName) {
const coursePrompt = coursePrompts[courseName];
if (!coursePrompt) {
return {
coursePrompt: "Sorry, I could not find information on that course.",
};
} else {
return {
coursePrompt: `Here are the details for the ${courseName} course. Remember to maintain a friendly, encouraging tone and focus on how these features benefit the student: ${coursePrompt}`,
};
}
}
Functional Prompt Design
Since IDNZ has so many courses and related details, putting everything into a single prompt would have made it too heavy and less responsive, so the two-step API approach seemed best to me. In this approach, the assistant initially only has access to course names within the prompt. If a user expresses interest in a particular course, the AI triggers a function call that retrieves full details for that specific course through a server action function that plucks relevant information from a JSON file. This makes the assistant speedy and efficient, reducing token usage as it doesn't carry a massive load of information right from the start.
Hubspot Integration
IDNZ wanted the chatbot to work alongside their existing HubSpot processes. Typically, interest was registered via a form on the website, which funneled personal data into HubSpot. I integrated the chatbot with HubSpot's REST API so that it would prompt the user for their name and email address, then immediately send an API request to HubSpot—automating the lead capture process. There was some debate about whether or not to gate the chatbot conversation behind a required name and email input, since that could affect engagement. However, in the end, the team decided that guaranteed lead capture was crucial, so we retained that prerequisite screen.
SquareSpace Code Injection
Because their main website was Squarespace-based, I wrote the assistant in such a way that it could be injected with a single line of code through an iframe. This required building event listeners capable of sending messages between the iframe and the main Squarespace page to handle resizing and toggling using the Window.postMessage() API with proper origin validation. When users click on the chatbot, it sends a message to the window to expand or contract both the containing div and the chatbot interface itself through a series of CSS transitions managed by React's useEffect hooks. This setup simplifies the client's deployment since they only need to paste one snippet into their pages without worrying about complex installations.
Embed file
A big part of this project was ensuring a balance between complexity and ease of use. And in order to implement the Squarespace injection idea, I ended up creating an embedding file that initializes a widget on the Squarespace site at an absolute location, which calls on the chatbot's Next.js application from its original hosting source. By implementing this embedding file, I preserved all the customization benefits of our personalized chatbot—rather than forcing us into more costly and restrictive third-party Squarespace-integrated chat systems. This architecture also made updates and maintenance far simpler while still giving IDNZ a robust AI assistant.
Technologies
- Next.js
- React
- OpenAI GPT-4
- HubSpot API
- TypeScript
- Node.js
- WebSockets
Project Gallery
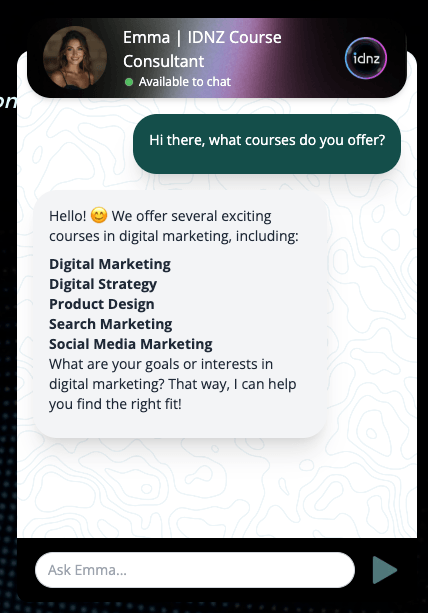
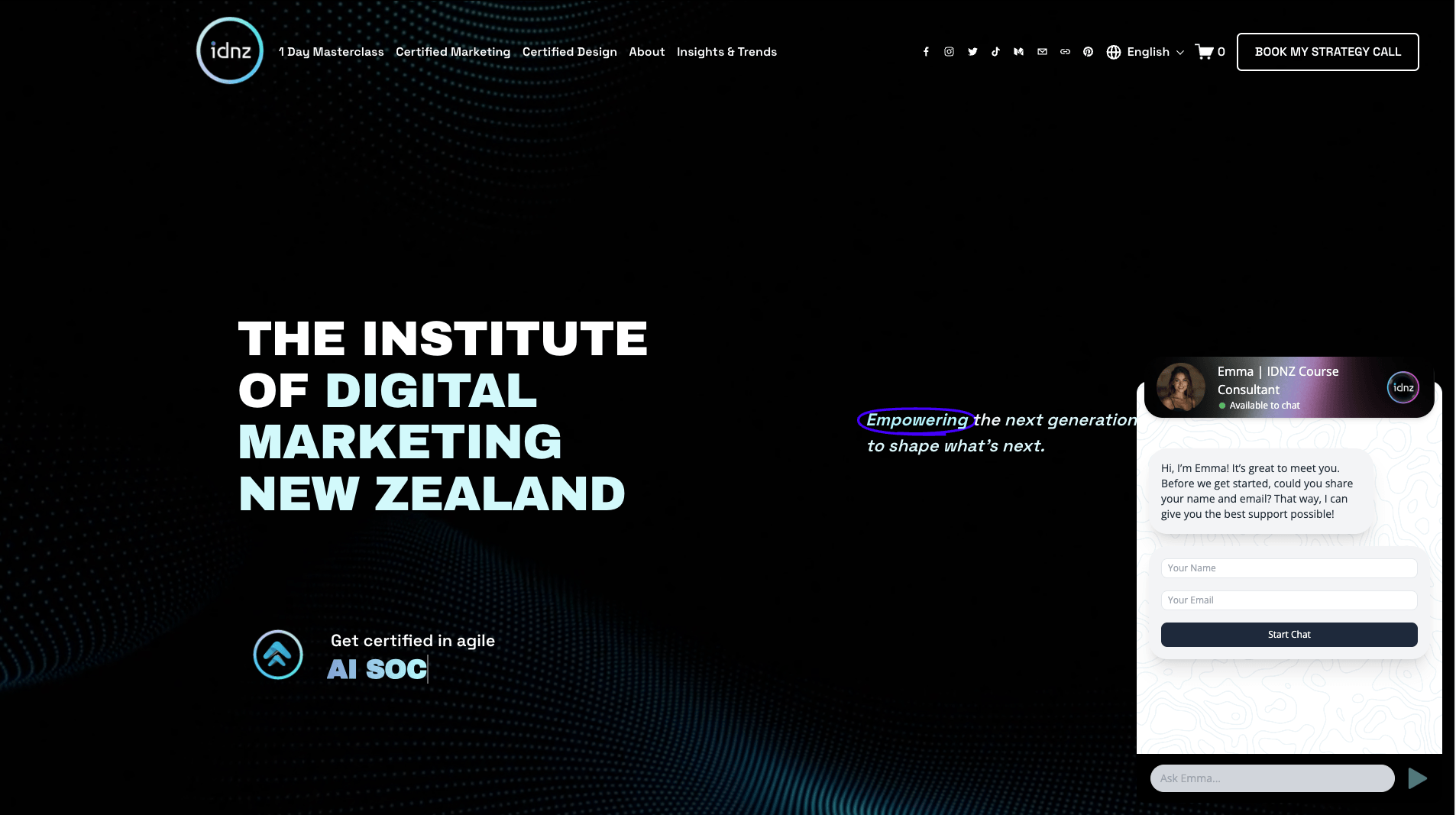
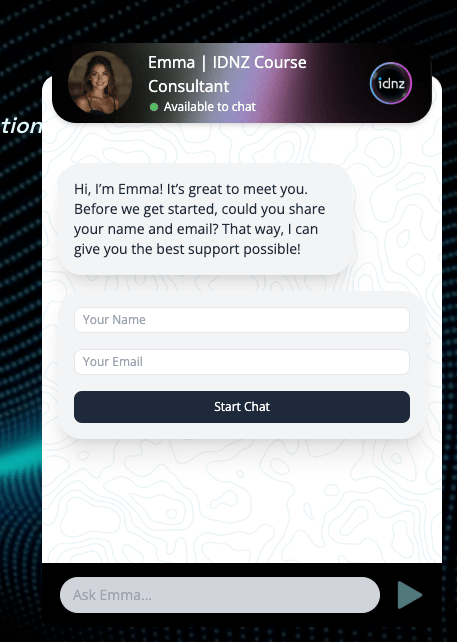