Digital Language Quest
Creating an online education platform, Digital Language Quest, to provide teachers with programmatically generated educational resources, including worksheets and games, that can be customized and shared with students. The platform utilizes AI, Google Docs, and Google Scripts to streamline the creation and distribution of resources, while ensuring security and access control.
Main Takeaways
- Implemented a multi-tier authentication system with role-based middleware protection for educational resources
- Designed a secure license key infrastructure for distributing and validating access to premium educational content
- Built a scalable architecture with separate admin, teacher, and student interfaces to manage educational resources
Introduction and Problem Statement
As a teacher, I recognized the challenge of creating engaging and effective educational resources, and the need for a platform that could provide teachers with easy access to customizable and shareable resources. Existing worksheet generators and educational platforms often lack AI implementation and provide limited functionality, leading to a gap in the market for a comprehensive solution. I decided to create Digital Language Quest to address this need and provide a platform for teachers to access and share educational resources. The platform was designed to be user-friendly, with a custom Next.js middleware that manages authentication flows for different user types (students, teachers, and administrators), ensuring appropriate access controls while maintaining an intuitive user experience regardless of technical proficiency.
Technical Implementation
I developed a Next.js app that followed App Router pattern with separate namespaced routes for games, admin interfaces, and authentication. The platform's authentication system is built on NextAuth.js with custom JWT token handling, including specialized callbacks for token and session management as evidenced in the middleware code. The authentication flow includes custom credential providers for students and Google OAuth integration for teachers, with dynamic route protection based on user roles stored in JWT tokens. The platform utilizes a Prisma ORM database to store user information, license keys, and usage data, with optimized database queries and connection pooling for production environments.
export async function middleware(request: NextRequest) {
// Get JWT token
const token = await getToken({ req: request })
const path = request.nextUrl.pathname
const isAuth = !!token
const isAuthPage = path.startsWith('/auth')
const isAdminPage = path.startsWith('/admin')
const isGamePath = path.startsWith('/games/')
// Game access check
if (isGamePath) {
if (!isAuth) {
console.log('Middleware: Unauthenticated user
attempting to access game')
return NextResponse.redirect(new URL('/auth/signin',
request.url))
}
Resource Generation and Sharing
The platform generates resources using a combination of client and server-side rendering strategies. Educational games are implemented as isolated modules with their own components, contexts, and utility functions. Each game includes specialized components for both student interaction and teacher customization options. Data flow is managed through React Context providers and custom hooks to maintain state across components. The system includes an API endpoint specifically for tracking resource generation metrics, which allows for usage monitoring and future feature planning based on popularity.
Security and Access Control
To ensure security and access control, I implemented a multi-layered approach centered on the custom middleware shown in the code. The middleware intercepts all non-static requests and performs token validation using NextAuth's getToken function. The access control system verifies not only authentication status but also specific resource permissions stored within the JWT token (as shown in the gameAccess array check). This allows for granular control over which teachers and students can access specific games and resources. The system includes specialized redirects based on user type, with different flows for students versus teachers, ensuring appropriate user experiences. Administrative routes are protected with an additional layer of authorization requiring an 'admin' accessLevel property on the token, preventing unauthorized access to sensitive operations like license key management.
Challenges and Solutions
One of the major challenges I faced was designing a secure and efficient license key system for managing access to premium educational resources. I solved this through a comprehensive API structure with dedicated endpoints for license key generation, validation, and management. The implementation includes external generation capabilities for integration with the Teachers Pay Teachers marketplace, allowing for automated access provisioning upon purchase. Another significant challenge was creating a unified authentication system that could accommodate both teacher and student users with different authentication flows and permission levels. I addressed this with a flexible NextAuth configuration using custom callbacks and providers, coupled with the middleware-based route protection system. This approach enables context-aware authentication checks that adjust based on the requesting user's role and the specific resource being accessed.
Problem-Solving and Iteration
Throughout the development process, I encountered and solved numerous technical challenges requiring creative solutions. One example was optimizing the route protection logic to minimize database queries while maintaining strict access controls. The middleware implementation demonstrates this approach by extracting all necessary permission data from the JWT token itself rather than making database calls on each request. Another key iteration was the development of specialized admin tools for license key management and system monitoring. These tools include interfaces for generating license keys in bulk, viewing access statistics, and managing user permissions. The project architecture evolved to support increasing complexity through careful organization of components, contexts, and utilities into logical namespaces, allowing for maintainable code despite the diverse feature set spanning authentication, resource generation, and educational games.
Technologies
- Next.js
- TypeScript
- NextAuth.js
- Prisma
- JWT
- Google OAuth
- API Routes
- Middleware
Project Gallery
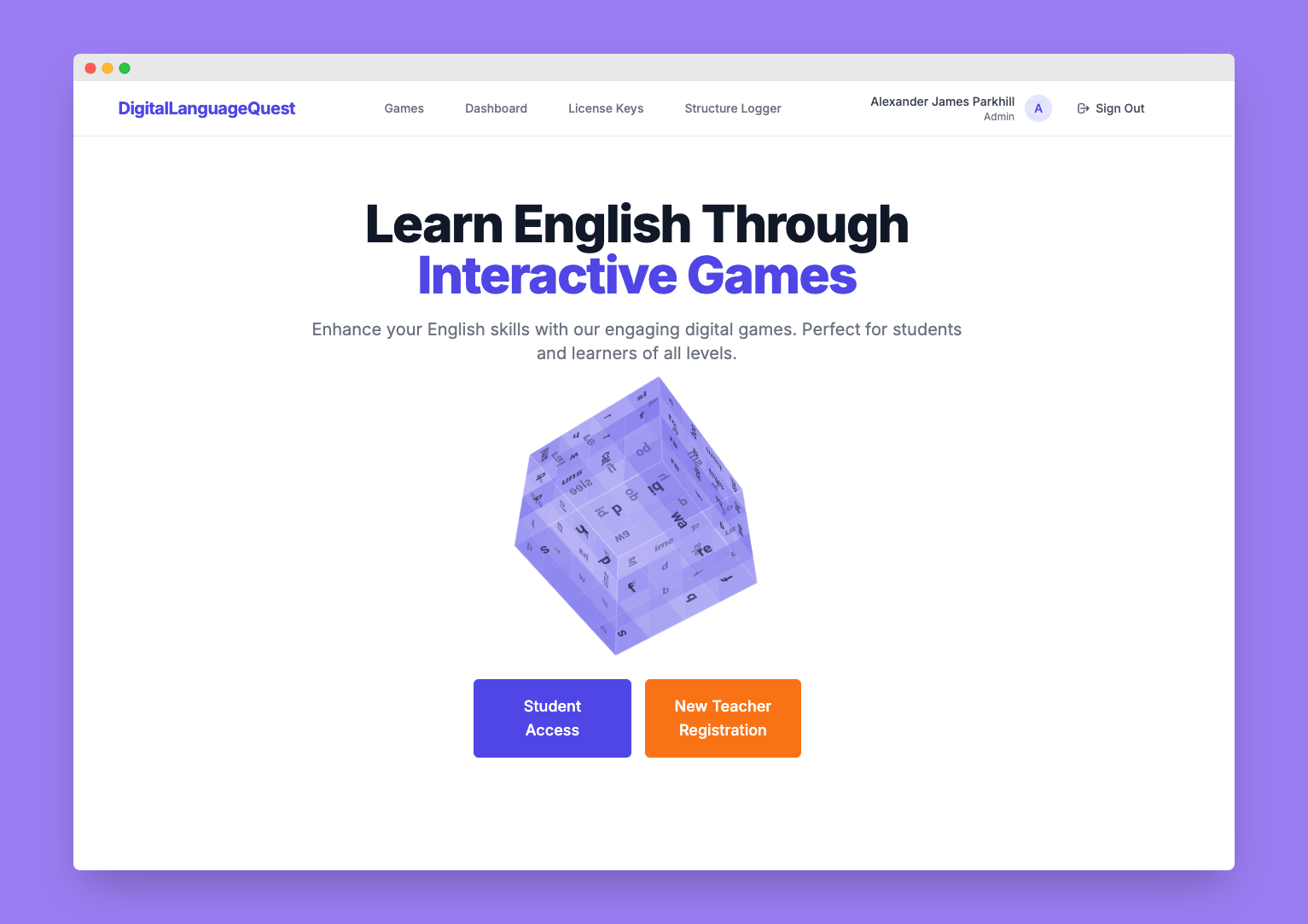
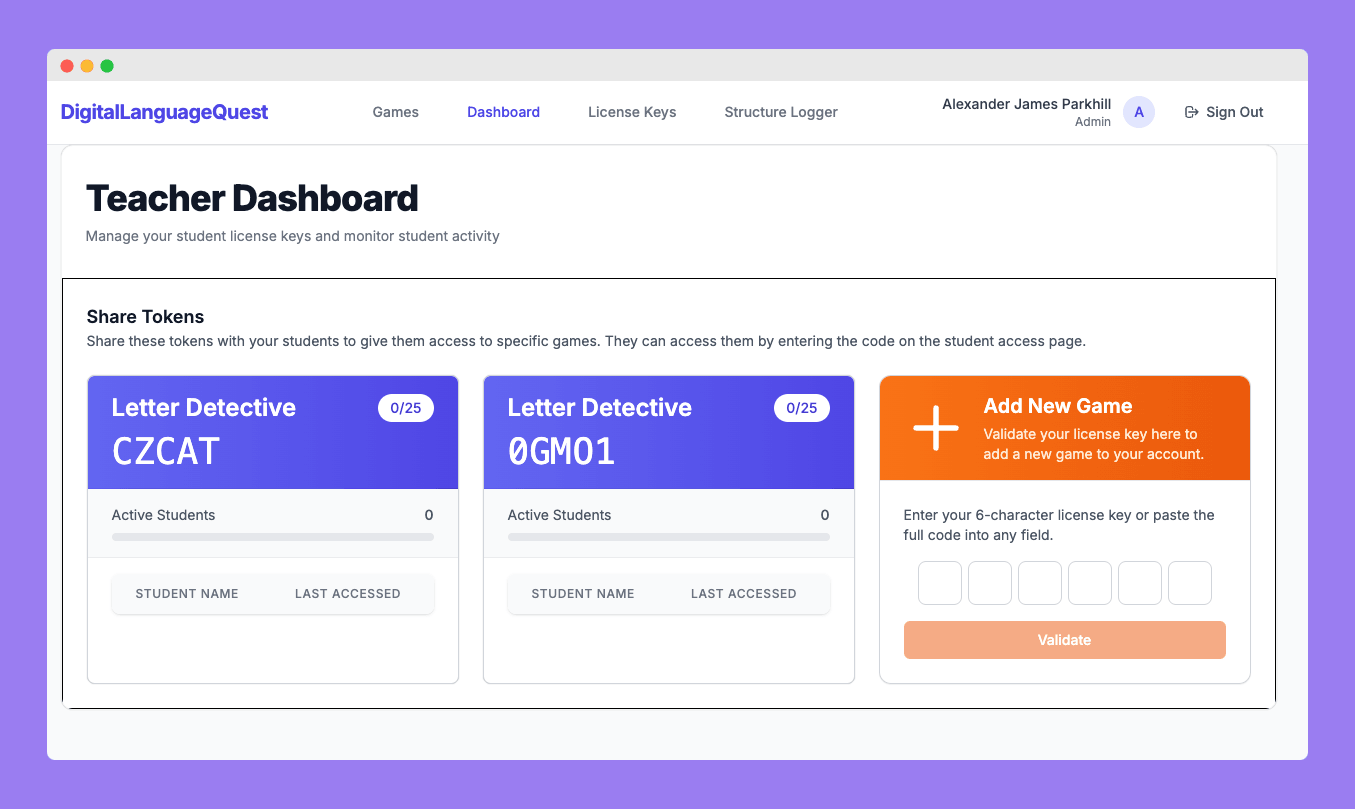
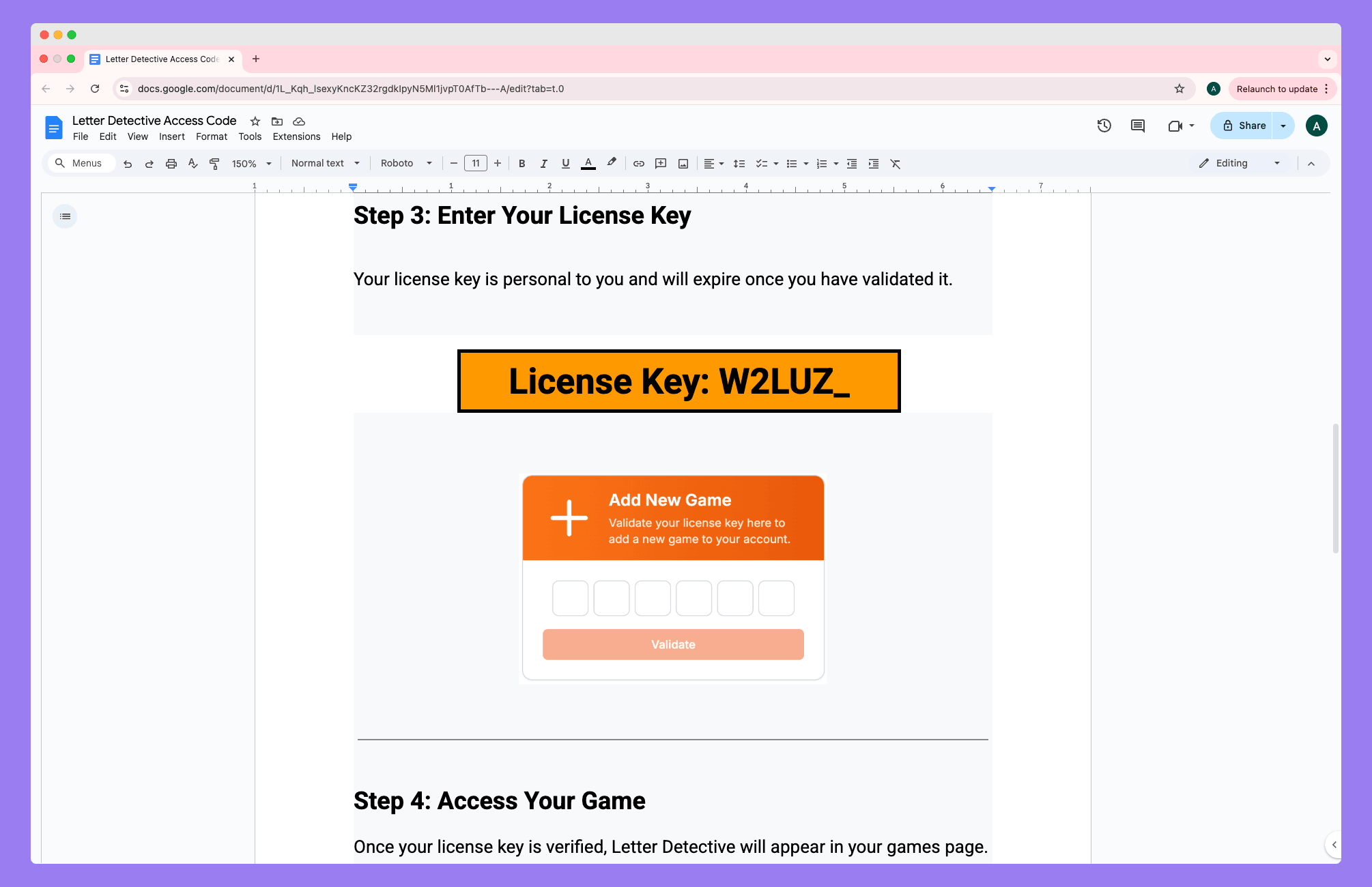
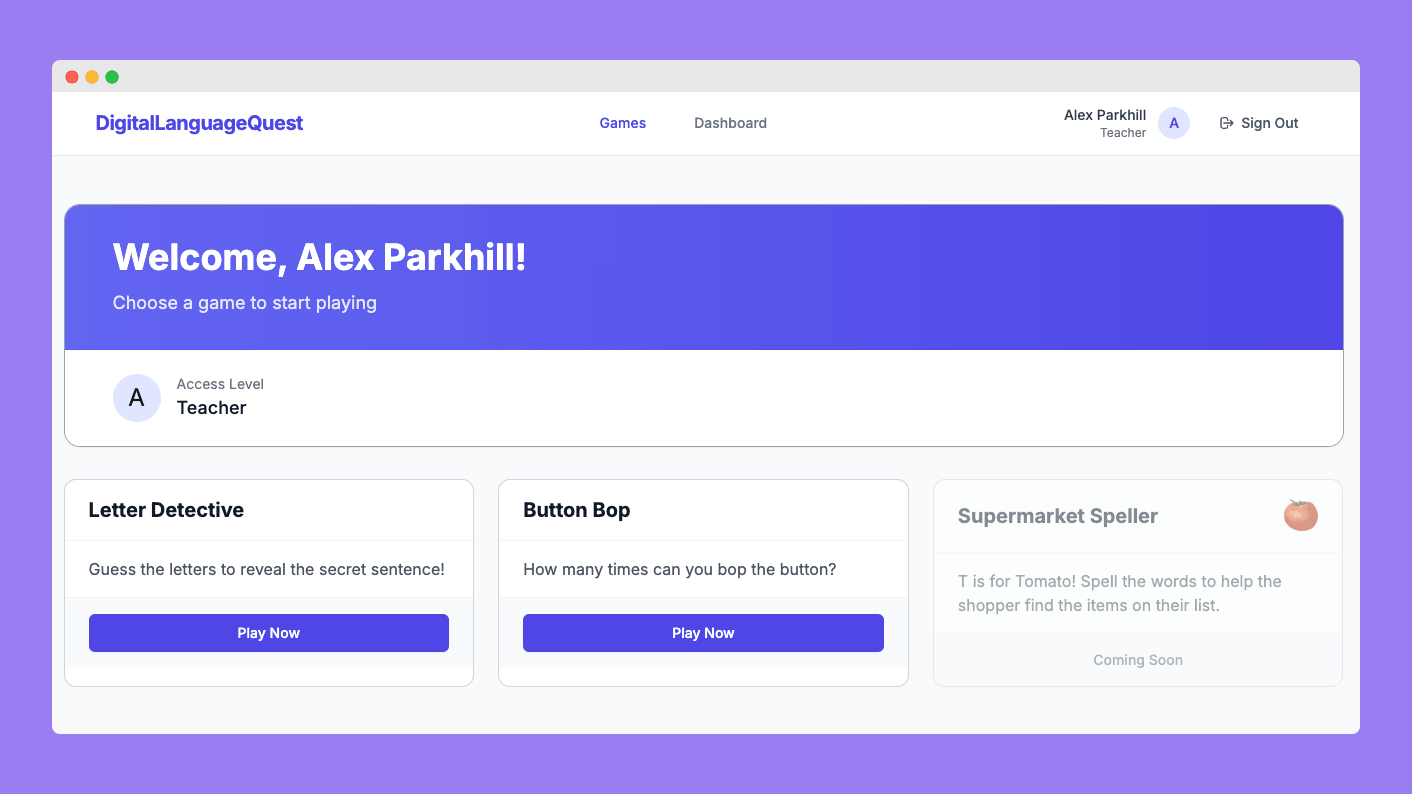
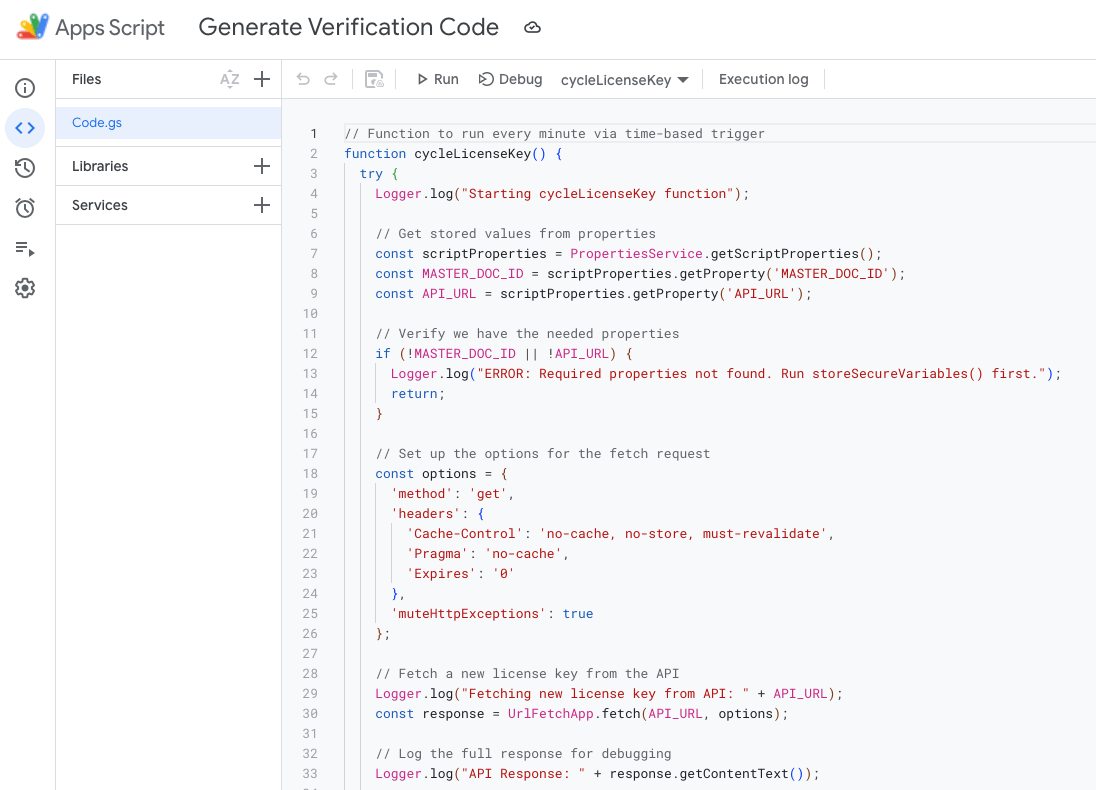
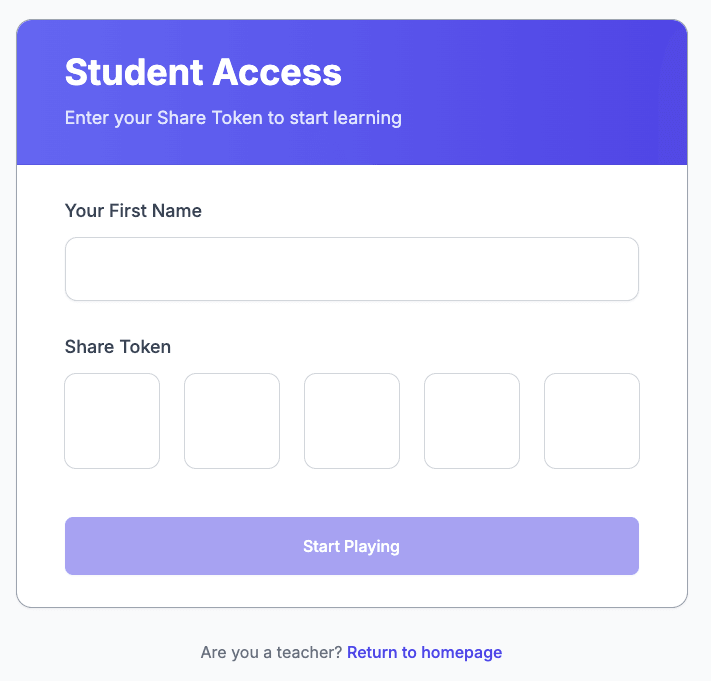